はじめに
今回は、Flutterでテーブルを作る方法について紹介します。
最近はWindowsアプリを作っているので、AndroidではなくWindowsアプリとして作成してみます。
Flutterでテーブルを作るために一番手軽なのが、DataTableウィジェットを使うことです。
今回はDataTableの使い方について解説してきます。
基本的なテーブル
まずは、一番シンプルな構成のテーブルを作ってみます。
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
// ここでDataTableを設定
child: DataTable(
// まずはカラム(列のヘッダー)を作成 -> columns配列の中にDataColumnウィジェットを定義
columns: [
DataColumn(label: Text('test1')),
DataColumn(label: Text('test2')),
],
// 行を作成
rows: [
// 1行目
DataRow(
cells: [
// 1行目の1つ目のデータ
DataCell(Text('content1-1')),
// 1行目の2つ目のデータ
DataCell(Text('content1-2')),
]
),
// 2行目
DataRow(
cells: [
DataCell(Text('content2-1')),
DataCell(Text('content2-2')),
]
),
// 3行目
DataRow(
cells: [
DataCell(Text('content3-1')),
DataCell(Text('content3-2')),
]
),
],
)
),
);
}
}
これでシンプルなテーブルが完成しました。
注意点としては、カラム数と行のデータ数が一致していないとエラーになる点です。
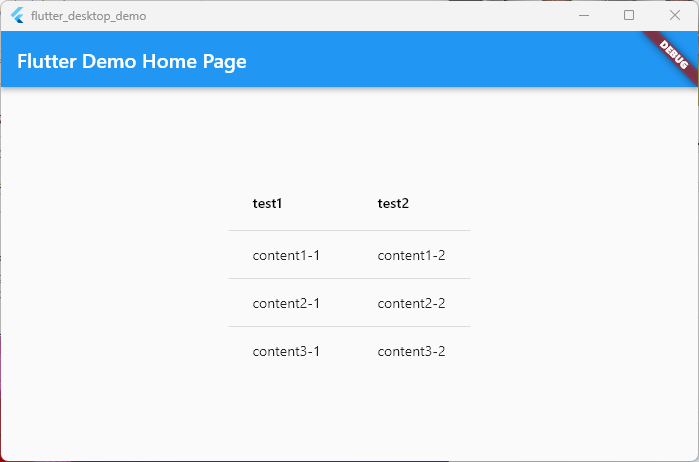
デザインを修正
次は、一番大事なとこですね。
今のままだとかなりシンプルなデザインなので自分の好みのデザインに変えてみましょう。
全体像(カスタムバージョン)
まずはカスタムしまくったやつをみてください!
これが全体像になります。
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: DataTable(
headingRowColor: MaterialStateProperty.all(Colors.green),
headingTextStyle: const TextStyle(
// 文字サイズ
fontSize: 70,
// 文字色
color: Colors.white,
// 背景色
backgroundColor: Colors.green,
// はみ出た文字をどうするか
overflow: TextOverflow.fade,
),
// ヘッダーの高さ(0にしたらヘッダーが消えます)
headingRowHeight: 72,
// 枠線
border: TableBorder.all(width: 1, color: Colors.white),
// データ間のスペース
columnSpacing: 30,
// デコレーション
decoration: BoxDecoration(
// グラデーション(colorsに入れた色のグラデーションが出来る)
gradient: const LinearGradient(colors: [Colors.red, Colors.blue, Colors.yellow]),
// 丸みをつける
borderRadius: BorderRadius.circular(30),
),
dataTextStyle: const TextStyle(fontSize: 20),
columns: const [
DataColumn(label: Text('test1'),),
DataColumn(label: Text('test2'),),
],
// 行を作成
rows: const [
// 1行目
DataRow(
cells: [
// 1行目の1つ目のデータ
DataCell(
// 中央揃え
Center(child: Text('content1-1'),)
),
// 1行目の2つ目のデータ
DataCell(
Center(child: Text('content1-2'),)
),
]
),
// 2行目
DataRow(
cells: [
DataCell(Text('content2-1')),
DataCell(Text('content2-2')),
]
),
// 3行目
DataRow(
cells: [
DataCell(Text('content3-1')),
DataCell(Text('content3-2')),
]
),
],
)
),
);
}
}
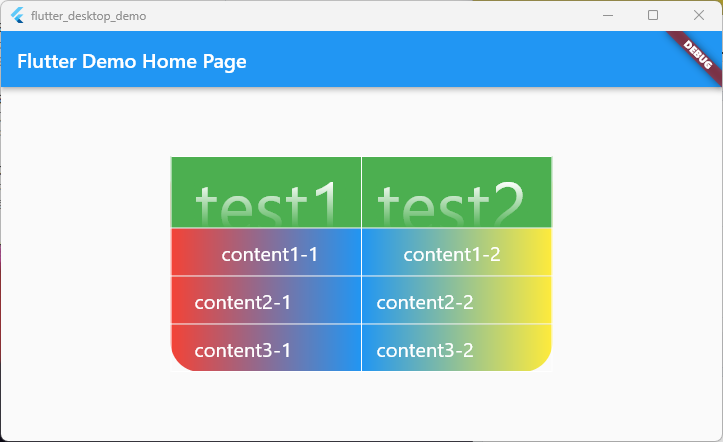
ヘッダーのデザイン変更
次は1つずつデザイン変更について解説していきます。
まず、ヘッダーのデザインを変更するには、DataTableのプロパティを変更します。
// ヘッダーの背景色
headingRowColor: MaterialStateProperty.all(Colors.green),
headingTextStyle: const TextStyle(
// 文字サイズ
fontSize: 70,
// 文字色
color: Colors.white,
// はみ出た文字をどうするか
overflow: TextOverflow.fade,
),
// ヘッダーの高さ(0にしたらヘッダーが消えます)
headingRowHeight: 72,
DataTableのプロパティを変更することでテーブルに対して共通の設定が可能になります。
1つ1つ設定したい場合は、columnsの中に設定を入れます。
行のデザイン変更
行のデザインを変更する際もDataTableのプロパティを変更します。
// 枠線
border: TableBorder.all(width: 1, color: Colors.white),
// データ間のスペース
columnSpacing: 30,
// デコレーション
decoration: BoxDecoration(
// グラデーション(colorsに入れた色のグラデーションが出来る)
gradient: const LinearGradient(colors: [Colors.red, Colors.blue, Colors.yellow]),
// 丸みをつける
borderRadius: BorderRadius.circular(30),
),
dataTextStyle: const TextStyle(fontSize: 20),
グラデーションもLinearGradient以外にもあるので、試してみます!
gradient: const RadialGradient(colors: [Colors.red, Colors.black54, Colors.amber]),
このように、RadialGradientにすると、下のようになります。
面白いですね!色の変更をして遊んでみてください!
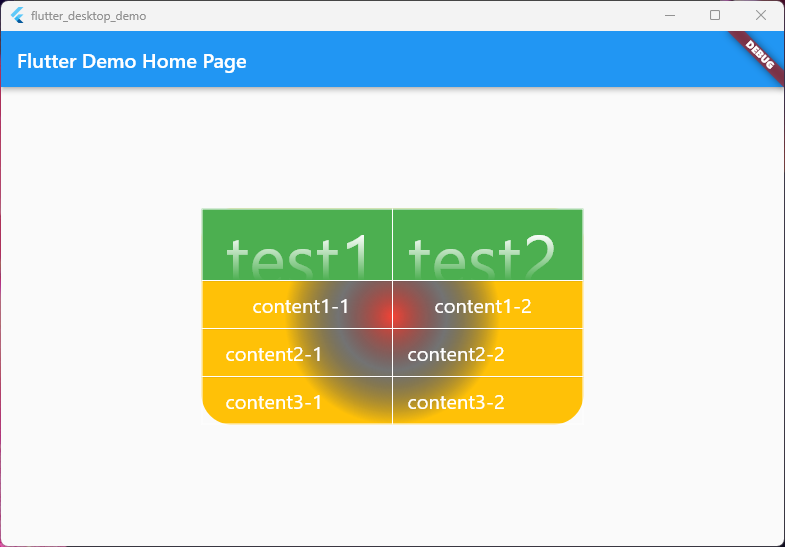
データの追加
次は、動的にデータを変化させたい場合はどうするのかを見ていきます。
今回はFlotingActionButtonを押したときに1行増やしてみたいと思います。
///
~ 変更はないので省略 ~
///
class _MyHomePageState extends State<MyHomePage> {
// 初期値として既に入っているデータを準備
// このように変数にしておくと、ボタンの操作等でデータを追加したいときや削除したいときに便利
List<DataRow> rows = [
const DataRow(
cells: [
DataCell(Center(child: Text('content1-1'),)),
DataCell(Center(child: Text('content1-2'),)),
]
),
const DataRow(
cells: [
DataCell(Text('content2-1')),
DataCell(Text('content2-2')),
]
),
const DataRow(
cells: [
DataCell(Text('content3-1')),
DataCell(Text('content3-2')),
]
),
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: DataTable(
columns: const [
DataColumn(label: Center(child: Text('test1'),)),
DataColumn(label: Text('test2'),),
],
// 事前に定義しておいた変数を使用
rows: rows
)
),
floatingActionButton: FloatingActionButton(
child: const Icon(Icons.add),
onPressed: () {
// ★これ重要(値の変更を検知して画面に伝えてくれる)
// これがないと描画されずに、内部的にしかデータが増えない
setState(() {
// データを追加
rows.add(
const DataRow(
cells: [
DataCell(Text('newData1')),
DataCell(Text('newData2')),
]
)
);
});
},
),
);
}
}
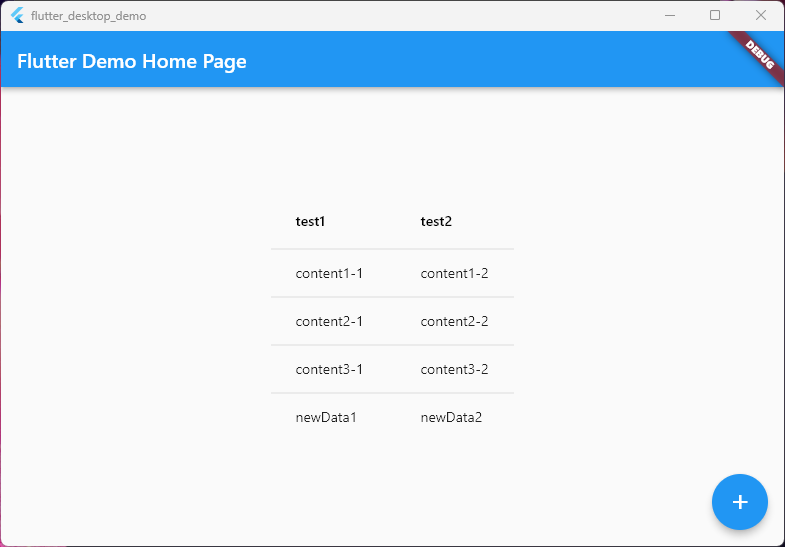
これで、データの追加も可能です。
DBから取得したデータも変数に入れておけば、簡単に扱うことができますね。
最後に
今回は、DataTableについて紹介をしました。
Androidアプリでは、画面サイズの関係であまり使用頻度は高くないかもしれませんが、
Windowsアプリでは使えそうですね!
ここまで読んでいただきありがとうございます。
コメント